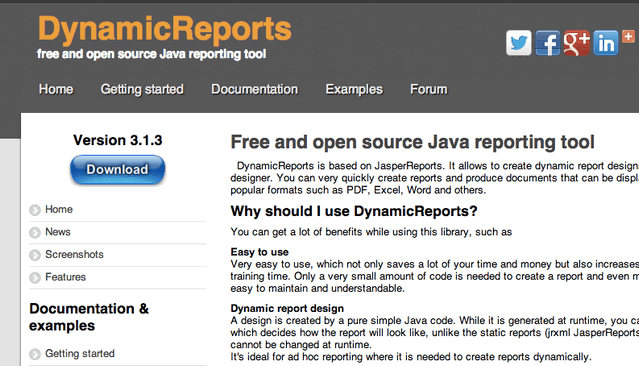
This example shows how to generate a simple report using DynamicReports and JasperReports. DynamicReports is a Java reporting library that allows you to produce report documents that can be exported into many popular formats. It is based on the well-known JasperReports library.
Tools used in this article :
- DynamicReports 3.1.3
- JasperReports 5.0.4
- MySQL 5.5
- Maven 3
- JDK 1.6.0
1. Download DynamicReports
Download DynamicReports from official website. If you use Maven, you can download it from the Maven central repository. For Maven projects add the following configuration to pom.xml file:
pom.xml
Markup
<dependencies>
<!-- DynamicReports -->
<dependency>
<groupId>net.sourceforge.dynamicreports</groupId>
<artifactId>dynamicreports-core</artifactId>
<version>3.1.3</version>
</dependency>
<!-- MySQL database driver -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.25</version>
</dependency>
</dependencies>
2. Report data source
Connect to a MySQL database using a JDBC driver.
Java
Class.forName("com.mysql.jdbc.Driver");
Connection connection =
DriverManager.getConnection("jdbc:mysql://hostname:port/dbname","username", "password");
3. Report builder
Let’s assume that the MySql server contains a database table customers and the customers table has the following structure:
Column name | Data type |
id | int |
first_name | varchar(50) |
last_name | varchar(50) |
date | date |
We will create a report that will get all customers from the table and the data will be put into the report.
3.1 Create a new empty report object.
Java
JasperReportBuilder report = DynamicReports.report();
3.2 Now create a report colum for each database column.
Java
Columns.column("Customer Id", "id", DataTypes.integerType())
The first parameter is a column label that will be shown in the report header.
The second parameter is a column name, this name should be the same as the database column name.
The third parameter is a Java type of the column.
The second parameter is a column name, this name should be the same as the database column name.
The third parameter is a Java type of the column.
3.3 Add the report columns.
Java
report
.columns(
Columns.column("Customer Id", "id", DataTypes.integerType()),
Columns.column("First Name", "first_name", DataTypes.stringType()),
Columns.column("Last Name", "last_name", DataTypes.stringType()),
Columns.column("Date", "date", DataTypes.dateType()))
3.4 Add a title text and a page number to the report.
Java
.title(//title of the report
Components.text("SimpleReportExample")
.setHorizontalAlignment(HorizontalAlignment.CENTER))
.pageFooter(Components.pageXofY())//show page number on the page footer
3.5 Finally set the data source query and connection.
Java
.setDataSource("SELECT id, first_name, last_name, date FROM customers ", connection);
4. Full Example
SimpleReportExample.java
Java
package net.sf.dynamicreports.examples;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import net.sf.dynamicreports.jasper.builder.JasperReportBuilder;
import net.sf.dynamicreports.report.builder.DynamicReports;
import net.sf.dynamicreports.report.builder.column.Columns;
import net.sf.dynamicreports.report.builder.component.Components;
import net.sf.dynamicreports.report.builder.datatype.DataTypes;
import net.sf.dynamicreports.report.constant.HorizontalAlignment;
import net.sf.dynamicreports.report.exception.DRException;
public class SimpleReportExample {
public static void main(String[] args) {
Connection connection = null;
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection(
"jdbc:mysql://hostname:port/dbname","username", "password");
} catch (SQLException e) {
e.printStackTrace();
return;
} catch (ClassNotFoundException e) {
e.printStackTrace();
return;
}
JasperReportBuilder report = DynamicReports.report();//a new report
report
.columns(
Columns.column("Customer Id", "id", DataTypes.integerType()),
Columns.column("First Name", "first_name", DataTypes.stringType()),
Columns.column("Last Name", "last_name", DataTypes.stringType()),
Columns.column("Date", "date", DataTypes.dateType()))
.title(//title of the report
Components.text("SimpleReportExample")
.setHorizontalAlignment(HorizontalAlignment.CENTER))
.pageFooter(Components.pageXofY())//show page number on the page footer
.setDataSource("SELECT id, first_name, last_name, date FROM customers",
connection);
try {
//show the report
report.show();
//export the report to a pdf file
report.toPdf(new FileOutputStream("c:/report.pdf"));
} catch (DRException e) {
e.printStackTrace();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
5. Run it
Run the SimpleReportExample class.
Figure : A pdf file will be created.
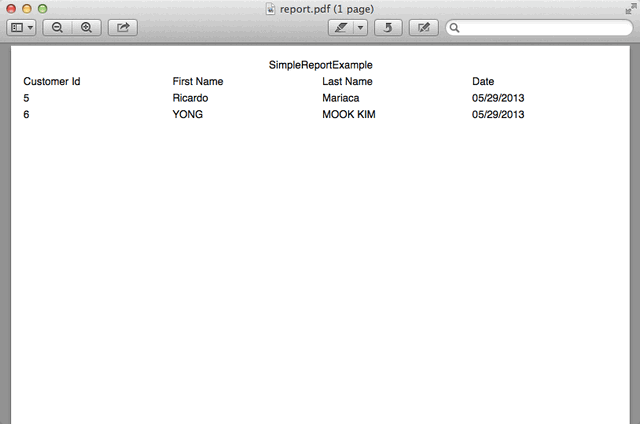
Download Source Code
Download it – JavaDynamicReports-Example.zip (11 KB)
No hay comentarios:
Publicar un comentario